Manuale:Sviluppo estensioni
Se si intende distribuire la propria estensione sui siti Wikimedia, leggere Writing an extension for deployment |
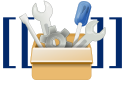
Ogni estensione consiste in tre parti:
Un'estensione minimale consisterà nella seguente struttura:
- MyExtension/extension.json
- Salva le istruzioni d'installazione. Il nome del file deve essere extension.json. (In versioni anteriori a MediaWiki 1.25, le istruzioni di installazione stavano in file
MyExtension/MyExtension.php
con il nome dell'estensione. Molte estensioni hanno ancora retro-compatibilità con questo file PHP.) - MyExtension/includes/ (or MyExtension/src/)
- Salva il codice PHP di esecuzione dell'estensione.
- MyExtension/resources/ (or MyExtension/modules/)
- Salva le risorse del lato client come JavaScript, CSS e LESS per l'estensione.
- MyExtension/i18n/*.json
- Salva le informazioni di localizzazione dell'estensione.
Quando sviluppi un'estensione, sostituisci la scritta MyExtension con il nome della tua estensione. Usa nomi nella convenzione UpperCamelCase per la cartella esterna per i tuoi file PHP. Questa è la convenzione generale per i nomi dei file.[1] (Il BoilerPlate extension è un buon punto di partenza per la tua estensione.)
$wgMainCacheType = CACHE_NONE
e $wgCacheDirectory = false
, altrimenti i messaggi di sistema ed altre variazioni potrebbero non essere mostrate.
Installazione
L'obiettivo nella scrittura della porzione di impostazione è rendere l'installazione dell'estensione la più semplice possibile, e l'utente deve solo aggiungere questa linea a LocalSettings.php:
wfLoadExtension( 'MyExtension' );
Se vuoi rendere l'estensione configurabile all'utente, devi definire e documentare alcuni parametri di configurazione e la configurazione dell'utente dovrebbe assomigliare a questa:
wfLoadExtension( 'MyExtension' );
$wgMyExtensionConfigThis = 1;
$wgMyExtensionConfigThat = false;
Per raggiungere questa semplicità, il file di installazione deve svolgere una serie di attività (descritte in dettaglio nelle seguenti sezioni):
- registrare qualsiasi gestore multimediale, funzione parser, pagina speciale, etichetta XML personalizzata e variabili utilizzate dalla tua estensione.
- definire e/o validare ogni configurazione variabili che hai definito nell'estensione.
- preparare le classi utilizzate dalla tua estensione per il caricamento automatico
- determinare quali parti della configurazione devono essere eseguite immediatamente e quali devono essere rimandate fino a quando il nucleo di MediaWiki non è stato inizializzato e configurato
- definire ogni ulteriore hooks necessario per l'estensione
- creare o controllare ogni nuova tabella di database richiesta dalla tua estensione.
- impostare la localizzazione per l'estensione
.md
. For example, see the README.md
file for Parsoid on Phabricator Diffusion.
Registrare funzionalità con MediaWiki
MediaWiki elenca tutte le estensioni che sono state installate nella sua pagina Special:Version
.
Per esempio, è possibile tutte le estensioni installate in questo wiki in Special:Version.
Versione MediaWiki: | ≥ 1.25 |
Per fare questo, aggiungere i dettagli dell'estensione a extension.json . Il risultato finale dovrebbe apparire più o meno così:
{
"name": "Example",
"author": "John Doe",
"url": "https://www.mediawiki.org/wiki/Extension:Example",
"description": "This extension is an example and performs no discernible function",
"version": "1.5",
"license-name": "GPL-2.0-or-later",
"type": "validextensionclass",
"manifest_version": 1
}
Molti campi sono facoltativi, ma è comunque una buona norma compilarli.
Il manifest_version
si riferisce alla versione dello schema su cui è scritto il file extension.json .
Le versioni disponibili sono 1 e 2. Vedere qui per la documentazione su questa funzionalità.
A meno che non sia necessario supportare una versione precedente di MediaWiki, scegliere la versione più recente.
Oltre alla registrazione di cui sopra, è necessario "agganciare" la propria funzione a MediaWiki. Quanto sopra configura solo la pagina Special:Version. Il modo per farlo dipende dal tipo di estensione. Per i dettagli, si prega di consultare la documentazione per ogni tipo di estensione:
Rendere l'estensione configurabile dall'utente
Se si desidera che l'utente sia in grado di configurare l'estensione, è necessario provvedere una o più variabili di configurazione. È buona norma dare un nome univoco a tali variabili. Queste dovrebbero anche seguire le convenzioni nomi di MediaWiki (e.g. le variabili globali devono iniziare con $wg).
Ad esempio, se l'estensione si chiama "MyExtension", si consiglia di dare a tutte le variabili di configurazione un nome che inizi con $wgMyExtension
.
È importante che nulla del nucleo di MediaWiki inizalizzi le sue variabili in questo modo e che si sia fatto un ragionevole lavoro di controllo per verificare che nessuna delle estensioni pubblicate inizalizzi le proprie variabili in questo modo.
Gli utenti non accetteranno di dover scegliere tra la tua estensione e alcune altre estensioni perché i nomi di variabili scelte si sovrappongono ad altre esistenti.
È inoltre buona norma includere nelle note di installazione un'ampia documentazione delle variabili di configurazione.
Here is an example boiler plate that can be used to get started:
{
"name": "BoilerPlate",
"version": "0.0.0",
"author": [
"Your Name"
],
"url": "https://www.mediawiki.org/wiki/Extension:BoilerPlate",
"descriptionmsg": "boilerplate-desc",
"license-name": "GPL-2.0-or-later",
"type": "other",
"AutoloadClasses": {
"BoilerPlateHooks": "includes/BoilerPlateHooks.php",
"SpecialHelloWorld": "includes/SpecialHelloWorld.php"
},
"config": {
"BoilerPlateEnableFoo": {
"value": true,
"description": "Enables the foo functionality"
}
},
"callback": "BoilerPlateHooks::onExtensionLoad",
"ExtensionMessagesFiles": {
"BoilerPlateAlias": "BoilerPlate.i18n.alias.php"
},
"Hooks": {
"NameOfHook": "BoilerPlateHooks::onNameOfHook"
},
"MessagesDirs": {
"BoilerPlate": [
"i18n"
]
},
"ResourceModules": {
"ext.boilerPlate.foo": {
"scripts": [
"resources/ext.boilerPlate.js",
"resources/ext.boilerPlate.foo.js"
],
"styles": [
"resources/ext.boilerPlate.foo.css"
]
}
},
"ResourceFileModulePaths": {
"localBasePath": "",
"remoteExtPath": "BoilerPlate"
},
"SpecialPages": {
"HelloWorld": "SpecialHelloWorld"
},
"manifest_version": 2
}
Si noti che dopo aver chiamato wfLoadExtension( 'BoilerPlate' );
la variabile globale $wgBoilerPlateEnableFoo
non esiste.
Se si imposta la variabile, ad es. in LocalSettings.php
allora il valore predefinito dato in extension.json non sarà usato.
Per ulteriori dettagli su come utilizzare una variabile globale all'interno di una estensione personalizzata, si prega di fare riferimento a Manual:Configuration for developers .
Preparare le classi per il caricamento automatico
Se si sceglie di utilizzare le classi per implementare la propria estensione, MediaWiki fornisce un meccanismo semplificato per aiutare PHP a trovare il file sorgente in cui si trova la classe.
Nella maggior parte dei casi questo dovrebbe eliminare la necessità di scrivere il proprio metodo __autoload($classname)
.
Per utilizzare il meccanismo di autocaricamento di MediaWiki, si aggiungono voci al campo AutoloadClasses . La chiave di ogni elemento è il nome della classe; il valore è il file che contiene la definizione della classe. Per un'estensione semplice a una classe, di solito alla classe viene dato lo stesso nome dell'estensione, quindi la sezione di caricamento automatico potrebbe apparire come questa (l'estensione si chiama MyExtension):
{
"AutoloadClasses": {
"MyExtension": "includes/MyExtension.php"
}
}
Il nome del file è relativo alla directory in cui si trova il file extension.json.
Per estensioni più complesse, si deve considerare namespaces. Vedere Manual:Extension.json/Schema#AutoloadNamespaces per dettagli.
Definire hook addizionali
Vedere Manual:Hooks .
Aggiungere tabelle del database
Make sure the extension doesn't modify the core database tables. Instead, extension should create new tables with foreign keys to the relevant MW tables.
Se l'estensione ha bisogno di aggiungere le proprie tabelle del database, usare l'hook LoadExtensionSchemaUpdates . Vedere la pagina del manuale per più informazioni sull'utilizzo.
Impostare la localizzazione
Vedi:
Aggiungere registri
Su MediaWiki, tutte le azioni degli utenti sul wiki sono tracciate per garantire trasparenza e collaborazione. Vedere Manual:Logging to Special:Log per istruzioni.
Handling dependencies
Assume that an extension requires the presence of another extension, for example because functionalities or database tables are to be used and error messages are to be avoided in case of non-existence.
For example the extension CountingMarker requires the presence of the extension HitCounters for certain functions.
One way to specify this would be by using the requires
key in extension.json.
Another option is using ExtensionRegistry (available since MW 1.25):
if ( ExtensionRegistry::getInstance()->isLoaded( 'HitCounters', '>=1.1' ) {
/* do some extra stuff, if extension HitCounters is present in version 1.1 and above */
}
Currently (as of February 2024, MediaWiki 1.41.0) the name of the extension-to-be-checked needs to exactly match the name in their extension.json.[2][3]
Example: if you want to check the load status of extension "OpenIDConnect", you have to use it with a space
if ( ExtensionRegistry::getInstance()->isLoaded( 'OpenID Connect' ) {
...
}
Localizzazione
|
$wgMainCacheType = CACHE_NONE
e $wgCacheDirectory = false
, altrimenti le modifiche ai messaggi di sistema potrebbero non essere visualizzate.Se si vuole che l'estensione venga utilizzata su wiki che hanno un pubblico multilingue, è necessario aggiungere il supporto per la localizzazione alla propria estensione.
Archiviare messaggi in <language-key>.json
Archivia le definizioni dei messaggi in un file JSON di localizzazione, uno per ogni chiave linguistica in cui l'estensione è tradotta. I messaggi vengono salvati con una chiave messaggio e il messaggio stesso utilizzando il formato standard JSON. Ogni id del messaggio deve essere minuscolo e non può contenere spazi. Ogni chiave deve iniziare con l'estensione del nome in minuscolo. Un esempio si può trovare nell'estensione MobileFrontend. Ecco un esempio di file JSON minimale (in questo caso en.json):
en.json
{
"myextension-desc": "Adds the MyExtension great functionality.",
"myextension-action-message": "This is a test message"
}
Archiviare la documentazione messaggi in qqq.json
La documentazione per le chiavi dei messaggi può essere memorizzata nel file JSON per lo pseudolinguaggio con il codice qqq. Una documentazione dell'esempio sopra può essere:
qqq.json:
{
"myextension-desc": "The description of MyExtension used in Extension credits.",
"myextension-action-message": "Adds 'message' after 'action' triggered by user."
}
Caricare il file di localizzazione
Nel file extension.json, definire la posizione dei file dei messaggi (ad esempio, nella cartella i18n/):
{
"MessagesDirs": {
"MyExtension": [
"i18n"
]
}
}
Uso di wfMessage in PHP
Nel codice di configurazione e implementazione, sostituire ogni uso letterale del messaggio con una chiamata a wfMessage( $msgID, $param1, $param2, ... )
.
Nelle classi che implementa IContextSource (come pure in certe altre sottoclassi di SpecialPage), si può invece utilizzare $this->msg( $msgID, $param1, $param2, ... )
.
Esempio:
wfMessage( 'myextension-addition', '1', '2', '3' )->parse()
Uso di mw.message in JavaScript
È possibile utilizzare le funzioni di internazionalizzazione anche in JavaScript. Vedi Manual:Messages API per i dettagli.
Tipi di estensione
Le estensioni possono essere classificate in base alle tecniche di programmazione utilizzate per ottenere il loro effetto. La maggior parte delle estensioni complesse utilizzerà più di una di queste tecniche:
- Sottoclassificazione: MediaWiki si aspetta che alcuni tipi di estensioni siano implementate come sottoclassi di una classe base fornita da MediaWiki:
- Special pages – Le sottoclassi della classe SpecialPage sono usate per costruire pagine il cui contenuto è generato dinamicamente usando una combinazione dello stato attuale del sistema, dei parametri inseriti dall'utente e delle interrogazioni del database. È possibile generare sia rapporti che moduli di inserimento dati. Vengono utilizzati sia per scopi di reporting che di amministrazione.
- Skins – Le skin modificano l'aspetto di MediaWiki alterando il codice che produce le pagine, sottoclassificando la classe SkinTemplate di MediaWiki.
- Hooks – Una tecnica per iniettare codice PHP personalizzato in punti chiave dell'elaborazione di MediaWiki. Essi sono ampiamente utilizzati dal parser di MediaWiki, il suo sistema di localizzazione, quello di gestione delle estensioni, e quello di manutenzione delle pagine.
- Associazioni di Tag-function – Etichetta di stile XML associati a una funzione php che produce codice HTML. Non è necessario limitarsi a formattare il testo all'interno dei tag. Non c'è nemmeno bisogno di visualizzarlo. Molte estensioni di etichette utilizzano il testo come parametri che guidano la generazione di HTML che incorporano oggetti di Google, moduli di inserimento dati, feed RSS, estratti di articoli wiki selezionati.
- Parole magiche – Una tecnica per mappare una serie di stringhe di testo wiki a un singolo id associato a una funzione. Sia variables che parser functions utilizzano questa tecnica. Tutto il testo mappato a quell'id verrà sostituito con il valore di ritorno della funzione. La mappatura tra le stringhe di testo e l'id è memorizzata nell'array $magicWords. L'interpretazione dell'id è un processo piuttosto complesso - per maggiori informazioni, vedere Manuale:Parole magiche .
- Variable – Le variabili sono un termine improprio. Sono pezzi di wikitext che assomigliano a modelli, ma che non hanno parametri e a cui sono stati assegnati dei valori precostituiti. I markup standard di wiki, come
{{PAGENAME}}
o{{SITENAME}}
, sono esempi di variabili. Il loro nome deriva dalla fonte del loro valore: una variabile php o qualcosa che potrebbe essere assegnato a una variabile, ad esempio una stringa, un numero, un'espressione o il valore di ritorno di una funzione. - Parser functions –
{{functionname: argument 1 | argument 2 | argument 3...}}
. Come le estensioni delle etichette, le funzioni di analisi elaborano gli argomenti e restituiscono un valore. Le estensioni delle etichette sono differenti, il risultato delle funzioni è il wikitexto.
- Variable – Le variabili sono un termine improprio. Sono pezzi di wikitext che assomigliano a modelli, ma che non hanno parametri e a cui sono stati assegnati dei valori precostituiti. I markup standard di wiki, come
- Moduli dell'API – è possibile aggiungere moduli personalizzati alle action API di MediaWiki, che possono essere invocati da JavaScript, bot o client di terze parti.
- Page content models – If you need to store data in formats other than wikitext, JSON, etc. then you can create a new ContentHandler .
Supporto per altre versioni core
Esistono due convenzioni diffuse per il supporto delle vecchie versioni del nucleo di MediaWiki:
- Master: il ramo master dell'estensione è compatibile con il maggior numero possibile di vecchie versioni di core. Questo comporta un onere di manutenzione (gli hack per la retrocompatibilità devono essere mantenuti a lungo e le modifiche all'estensione devono essere testate con diverse versioni di MediaWiki), ma i siti che utilizzano vecchie versioni di MediaWiki beneficiano delle funzionalità aggiunte di recente all'estensione.
- Rami di rilascio: i rami di rilascio dell'estensione sono compatibili con i rami corrispondenti del nucleo, ad esempio i siti che usano MediaWiki 1.42 devono usare il ramo REL1_42 dell'estensione. (Per le estensioni ospitate su gerrit, questi rami vengono creati automaticamente quando vengono rilasciate nuove versioni di MediaWiki). Ciò si traduce in un codice più pulito e in uno sviluppo più rapido, ma gli utenti delle vecchie versioni del nucleo non beneficiano delle correzioni di bug e delle nuove funzionalità, a meno che non vengano ricompilati manualmente.
I manutentori delle estensioni dovrebbero dichiarare con il parametro compatibility policy
del template {{Estensione }} quale convenzione seguono.
Licenza
MediaWiki is an open-source project and users are encouraged to make any MediaWiki extensions under an Open Source Initiative (OSI) approved license compatible with GPL-2.0-or-later (Wikimedia's standard software license).
We recommend adopting one of the following compatible licenses for your projects in Gerrit:
- GNU General Public License, version 2 or later (GPL-2.0-or-later)
- MIT License (MIT)
- BSD License (BSD-3-Clause)
- Apache License 2.0 (Apache-2.0)
For extensions that have a compatible license, you can request developer access to the MediaWiki source repositories for extensions.
To specify the licence in code and with "license-name
" a key should be used to provide it's short name, e.g. "GPL-2.0-or-later" or "MIT" adhering to the list of identifiers at spdx.org.
Pubblicazione
Per autocategorizzare e standardizzare la documentazione dell'estensione esistente, vedere Template:Estensione . Per aggiungere la tua nuova estensione a questa Wiki:
A developer sharing their code in the MediaWiki code repository should expect:
- Feedback / Criticism / Code reviews
- Review and comments by other developers on things like framework use, security, efficiency and usability.
- Developer tweaking
- Other developers modifying your submission to improve or clean-up your code to meet new framework classes and methods, coding conventions and translations.
- Improved access for wiki sysadmins
- If you do decide to put your code on the wiki, another developer may decide to move it to the MediaWiki code repository for easier maintenance. You may then create a developer account to continue maintaining it.
- Future versions by other developers
- New branches of your code being created automatically as new versions of MediaWiki are released. You should backport to these branches if you want to support older versions.
- Incorporation of your code into other extensions with duplicate or similar purposes — incorporating the best features from each extension.
- Credit
- Credit for your work being preserved in future versions — including any merged extensions.
- Similarly, you should credit the developers of any extensions whose code you borrow from — especially when performing a merger.
Any developer who is uncomfortable with any of these actions occurring should not host in the code repository. You are still encouraged to create a summary page for your extension on the wiki to let people know about the extension, and where to download it.
Distribuzione e registrazione
Se si intende distribuire la propria estensione sui siti Wikimedia (compresa eventualmente Wikipedia), è necessario un ulteriore controllo in termini di prestazioni e sicurezza. Consultare Writing an extension for deployment .
Se l'estensione aggiunge dei namespace, si potrebbe voler registrare i suoi namespace predefiniti; allo stesso modo, se aggiungono tabelle o campi del database, si potrebbe volerli registrare a database field prefixes .
Si tenga presente che la revisione e la distribuzione di nuove estensioni sui siti Wikimedia può essere estremamente lenta e in alcuni casi ha richiesto più di due anni.[4]
Documentazione di aiuto
Dovreste fornire documentazione di aiuto di dominio pubblico per le funzioni fornite dalla vostra estensione.
The convention is for extensions to have their user-focused help pages under a pseudo-namespace of Help:Extension:<ExtensionName>
, with whatever subpages are required (the top level page will be automatically linked from the extension infobox if it exists).
Help:CirrusSearch è un buono esempio.
Si dovrebbe fornire agli utenti un link alla documentazione tramite la funzione addHelpLink() .
Releasing updates
There are a number of common approaches to releasing updates to extensions.
These are generally defined according to the compatibility policy of the extension (master
, rel
, or ltsrel
):
master
- Releases may be tagged with version numbers on the master branch, and documentation provided on the extension's homepage describing which extension versions are compatible with which core versions. Release branches will still be created automatically, and you may wish to delete these if they are not intended to be used.rel
andltsrel
- Release by backporting changes to theREL1_*
branches (either all changes, or only critical ones). Version numbers are generally not needed unless the extension is a dependency of another (the version number can then be provided in the other extension's configuration to ensure that incompatible combinations aren't installed). Many extensions will stay at the same version number for years.
Fornire supporto / collaborazione
Gli sviluppatori di estensioni devono aprire un account su Wikimedia Phabricator , e richiedere un nuovo progetto per l'estensione. Questo fornisce un luogo pubblico in cui gli utenti possono inviare problemi e suggerimenti e voi potete collaborare con gli utenti e gli altri sviluppatori per risolvere i bug e pianificare le funzionalità della vostra estensione.
Vedi anche
- Manual:Extension registration - provides further developer documentation on how to register extensions and skins.
- API:Extensions – spiega come l'estensione in grado di fornire una API per i client
- Manuale:Estensione wiki markup
- Manual:Coding conventions
- Best practices for extensions
- ResourceLoader
Learn by example
- Extension:Examples – implementa alcune funzioni di esempio con un'ampia documentazione in linea
- Extension:BoilerPlate – a functioning boilerplate extension, useful as a starting point for your own extension (repository git)
- Leggi l'estensione Example, basa il tuo codice sull'estensione BoilerPlate.
- cookiecutter-mediawiki-extension – a cookiecutter template which generates a boilerplate extension (with variables etc.)
- Permette di iniziare rapidamente a lavorare con la propria estensione.
- Può anche generare l'estensione Boilerplate.
- List of simple extensions - copiare il codice specifico da loro