Manual:Special pages
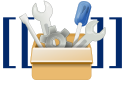
Special pages are pages that are created by the software on demand to perform a specific function.
For example, a special page might show all pages that have one or more links to an external site or it might create a form providing user submitted feedback.
Special pages are located in their own namespace (Special:) and are not editable directly like other pages. Developers can also create new special pages.
These pages by default are user-accessible and will generally show up in the list of all special pages at Special:SpecialPages. Some special pages are only accessible to users with certain permissions and accesses. Other special pages don't show up on the special page list at all and are only used by the wiki internally.
General Information
All built-in special pages that come with MediaWiki are called SpecialSomename.php
and are located in the includes/specials
directory.
Core special pages must be registered in the core list located in includes/specialpage/SpecialPageFactory.php
in order to be loaded by MediaWiki.
Special pages created by third party developers are generally stored in the extensions
directory in their own file or as part of a larger extension.
All special pages inherit from a class called SpecialPage which is defined in includes/specialpage/SpecialPage.php
.
When a new special page is created, the user rights needed to access the page can be defined.
These rights specify, among other things, whether the page will show up on Special:SpecialPages and whether the page is includable in other pages.
Special pages also have unique names that can be customized on a wiki. The general form is "Special:Pagename" where both "Special" and "Pagename" are customizable. The Special pseudo namespace can be translated in other languages. This translated namespace can be produced with the wikitext {{ns:special}}, on this wiki giving "Special". The name of the special page can also be redefined in a system message, for the site language, with the generic name of the special page as the ID.
A special page may or may not allow input. For example, Special:Export allows a user to define a specific page to export by calling Special:Export/Sun. If the special page allows complex input, additional parameters will be sent to the query string component of the URL for processing, e.g. https://www.mediawiki.org/w/index.php?title=Special:Recentchanges&days=3&limit=250.
- There are various ways to make special pages, but the one below is used by the bulk of official extensions , and adherence to this style is recommended. Also, be sure to include a credits block in the new special page for 'specialpage'. See
$wgExtensionCredits
for more details. - After making a new special page, be sure to add it to Category:Special page extensions so other people can find it.
- Special pages cannot be included within frames unless you use
$wgOut->allowClickjacking();
Basic special page template
MediaWiki 1.25 introduced a new way to load an extension. For older unsupported versions of MediaWiki, see an older revision. |
Most special page extensions require three files:
- Small setup file, which loads every time MediaWiki starts.
- File with the bulk of the code.
- Localisation file
MediaWiki coding conventions define the three files like this:
MyExtension/extension.json
- The setup file.MyExtension/includes/Special.php
- The special page code.i18n/*.json
- The localisation file .
Place all of the files in a new directory inside your MediaWiki extensions/
directory.
You should name the special page file after the extension. For example, Extension:Gadgets contains the file SpecialGadgets.php
.
If your extension uses more than one special page, you'll need more names.
In the example below, the special page's name is MyExtension.
After creating the files listed below, adding the following line to LocalSettings.php enables the extension:
wfLoadExtension( 'MyExtension' );
The setup file
Example setup file for MyExtension/extension.json
:
{
"name": "MyExtension",
"version": "0.0.0",
"author": [
"Your Name"
],
"url": "https://www.mediawiki.org/wiki/Extension:MyExtension",
"descriptionmsg": "myextension-desc",
"license-name": "MIT",
"type": "other",
"AutoloadNamespaces": {
"MediaWiki\\Extension\\MyExtension\\": "src/"
},
"SpecialPages": {
"MyExtension": "MediaWiki\\Extension\\MyExtension\\Special"
},
"MessagesDirs": {
"MyExtension": [
"i18n"
]
},
"manifest_version": 2,
"requires": {
"MediaWiki": ">= 1.40.0",
"platform": {
"php": ">= 5.6"
},
"extensions": {
"dependendExtension": "*"
}
}
}
This file registers several important and mandatory things:
- The location of the MediaWiki\Extension\MyExtension\Special class;
- The location of the localisation files;
- The new special page and its class name.
InvalidArgumentException: Provided specification is not an array
, it generally means that MediaWiki could not find the class specified in extension.json that implements your special page.The special page file
The body file (MyExtension/src/Special.php
) should contain a subclass of SpecialPage
or one of its subclasses.
This file loads automatically when someone requests the special page.
The example below implements the subclass SpecialMyExtension.
You need the __construct()
constructor because its first parameter names your special page.
execute()
is the main function called when a special page is accessed.
This function overrides the function SpecialPage::execute()
.
It passes the single parameter $par
, the subpage component of the current title.
For example, if someone follows a link to Special:MyExtension/blah, $par
contains "blah".
You should run Wikitext and HTML output through $wgOut
. Do not use 'print' or 'echo' directly when working within the wiki's user interface.
However, if you use your special page as an access point to custom XML or binary output, see Taking over output in your special page .
<?php
namespace MediaWiki\Extension\MyExtension;
class SpecialMyExtension extends \SpecialPage {
public function __construct() {
parent::__construct( 'MyExtension' );
}
public function execute( $par ) {
$request = $this->getRequest();
$output = $this->getOutput();
$this->setHeaders();
# Get request data from, e.g.
$param = $request->getText( 'param' );
# Do stuff
# ...
$wikitext = 'Hello world!';
$output->addWikiTextAsInterface( $wikitext );
}
}
The localisation file
- See Help:System message for how to get them translated.
All special pages specify a title, like 'My Extension'
.
- The title is used in the
<title>
and<h1>
elements of the extension's page and on Special:SpecialPages. - It can be anything, but should describe the special page and extension.
- It's specified through a message. The structure of the message is a key-value pair. The key,
'myextension'
, must be all lowercase.
An example of a localisation file in MyExtension/i18n/en.json
:
{
"@metadata": {
"authors": [
"<your username>"
]
},
"myextension": "My Extension",
"myextension-desc": "Adds the MyExtension functionality.",
"myextension-summary": "On this special page, do this simple thing and earn wonders.",
"group-myextensionrole": "Role of myextension",
"group-myextensionrole-member": "Member of role of myextension",
"grouppage-myextensionrole": "{{ns:project}}:Role of myextension",
"action-myextension": "XYZ doing.",
"right-myextension": "to do xyz"
}
In i18n/qqq.json
, the message documentation:
{
"@metadata": {
"authors": [
"<your username>"
]
},
"myextension": "The name of the extension's entry in Special:SpecialPages",
"myextension-desc": "{{desc}}",
"myextension-summary": "Description appearing on top of Special:MyExtension.",
"action-myextension": "{{doc-action|myextension}}",
"right-myextension": "{{doc-right|myextension}}"
}
Note that IDs should not start with an uppercase letter, and that a space in the ID should be written in the code as an underscore.
The -summary message is optional. It's created automatically by the parent class and shown on top of the special page, usually for a concise description of what the user can do on it. If you don't define its content, it will only be used when wiki administrators customize it on the wiki.
The aliases file
You can also internationalize the name of the special page by creating aliases for it. The example below uses the file "MyExtension.i18n.alias.php".
In this example, the special page MyExtension
registers an alias so the page becomes accessible at .../Special:My Extension
and .../Spezial:Meine_Erweiterung
in German.
Add your alias file to extension.json
:
...
"ExtensionMessagesFiles": {
"MyExtensionAlias": "MyExtension.i18n.alias.php"
},
...
Add special page aliases to MyExtension.i18n.alias.php
:
<?php
/**
* Aliases for myextension
*
* @file
* @ingroup Extensions
*/
$specialPageAliases = [];
/** English
* @author <your username>
*/
$specialPageAliases['en'] = [
'MyExtension' => [ 'MyExtension', 'My Extension' ],
];
/** Deutsch
* @author <your username>
*/
$specialPageAliases['de'] = [
'MyExtension' => [ 'MeineErweiterung', 'Meine Erweiterung' ],
];
Again, you should write a space in the ID and an underscore in the code.
For the page header and linking, the usual rules for page names apply.
If $wgCapitalLinks
is true, a lowercase letter is converted to uppercase, and an underscore is displayed as a space.
For example, instead of the above, we could use 'my_extension' => 'My extension'
, assuming we consistently identified the extension as my_extension
elsewhere.
Note that in the associative array for the English language, the string identifying our SpecialPage (MyExtension
in the example) is also a valid title.
Also note, the first element of $specialPageAliases['en']['MyExtension']
must be the same as the key ('MyExtension'
)! Otherwise Special:Specialpages will not list the page.
Special page group
You can set which group your special page appears under on Special:SpecialPages by overriding SpecialPage::getGroupName()
in your subclass.
/**
* Override the parent to set where the special page appears on Special:SpecialPages
* 'other' is the default. If that's what you want, you do not need to override.
* Specify 'media' to use the <code>specialpages-group-media</code> system interface message, which translates to 'Media reports and uploads' in English;
*
* @return string
*/
function getGroupName() {
return 'media';
}
Some common values are 'login', 'maintenance', 'media', 'other', 'pagetools', 'redirects', 'users'. You can see the accepted values at Special:AllMessages (search for specialpages-group) or browse the wiki using the pseudo language 'qqx' by going to Special:SpecialPages?uselang=qqx) and looking at the headings. Specify the word 'media' to use the interface message 'specialpages-group-media'.
If your special page doesn't fit into any of the preconfigured headings, you can add a new heading by adding it to your localisation file, see The localisation file).
The standard page groups that come with MediaWiki are listed in the localisation file. For example, the English messages are in languages/i18n/en.json
and begin with specialpages-group-
.
If you want to categorize your special page under users
, then the message is specialpages-group-users
.
The value for this key is the text that appears as the name of that category, for example, Users and rights
.
If your special page does not seem to fit under any of the existing categories, you can always make a new one.
In your extension's localisation file simply insert a new key for the messages
array.
In this example, we define the gamification
group:
{
"myextension": "My Extension",
"myextension-desc": "Adds the MyExtension functionality.",
"myextension-summary": "On this special page, do this simple thing and earn wonders",
"specialpages-group-gamification": "Gamification"
}
Now, assuming you set the return value for the method SpecialPage::getGroupName()
as gamification
in your class definition, reload Special:SpecialPages to see your new category.
Other Important Files
SpecialPage.php
Constructor
You can overload the constructor to initialize your own data, but the main reason you would want to do it is to change the behavior of the SpecialPage class itself. When you call the base class constructor from your child class, the following parameters are available:
function __construct( $name = '', $restriction = '', $listed = true );
- string
$name
Name of the special page, as seen in links and URLs - string
$restriction
User right required, e.g. "block" or "delete"; also see Restricting page access - boolean
$listed
Whether the page is listed in Special:Specialpages
SpecialPage->setHeaders()
This initialises the OutputPage object $wgOut
with the name and description of your special page.
It should always be called from your execute() method.
SpecialPage->getOutput()
This method returns an OutputPage object which can be accessed as described below. As in the example code, use
$output = $this->getOutput();
$output->addWikiTextAsInterface( 'Hello, World' );
instead of the deprecated $wgOut
global variable
SpecialPage->getRequest()
This method returns a WebRequest object which can be accessed as described below. As in the example code, use
$request = $this->getRequest();
$myparam = $request->getText( 'myparam' );
instead of the deprecated $wgRequest
global variable
SpecialPage->including()
Some special pages can be included from within another page. For example, if you add {{Special:RecentChanges}} to the wikitext of a page, it will insert a listing of recent changes within the existing content of the page.
Including a special page from another web page is only possible if you declared the page to be includable in the constructor.
You can do this by adding the following in the __construct()
method after the parent class initialization:
$this->mIncludable = true;
You can also define your special page class as extending the IncludableSpecialPage class.
The SpecialPage->including() function returns a boolean value telling you what context the special page is being called from: false if it is a separate web page, and true if it is being included from within another web page. Usually you will want to strip down the presentation somewhat if the page is being included.
SpecialPage->execute()
This is the function which your child class should overload.
It passes a single parameter, usually referred to cryptically as $par
(short for $parameter, as it is the parameter the users can feed to your special page).
This parameter is the subpage component of the current title.
For example, if someone follows a link to Special:MyExtension/blah, $par
will contain "blah".
Help page
MediaWiki version: | ≥ 1.25 |
It's useful to add help pages on MediaWiki.org, where they'll be translatable. To make sure users find your help page, it's advisable and very simple for your special page to link the help page in question:
$this->addHelpLink( 'Help:Extension:MyExtension' );
OutputPage.php
OutputPage.php contains the class definition for objects of type OutputPage
.
You can get an object of this class from your SpecialPage using
$output = $this->getOutput();
The variablename $output is, of course, arbitrary.
Whatever you call it, this is the variable you will use the most, because it is the way to send output to the browser (no, you don't use echo
or print
).
If you want to use it somewhere, declare the variable global:
function randomFunction() {
$output = $this->getOutput();
$output->addHTML( '<b>This is not a pipe...</b>' );
}
If you want to, you can create multiple OutputPage objects in different methods in your SpecialPage extension. They will add to the output in the order they are executed.
You can inspect the OutputPage class by viewing includes/OutputPage.php
(indeed, all of these can be inspected), but there are a few methods you should definitely know about.
OutputPage->addHTML()
Essentially the quick and dirty substitute for echo
.
It takes your input and adds it to the buffer: no questions asked.
In the below action, if $action
contains user-data, it could easily have XSS, evil stuff, or the spawn of Satan injected in.
You're better off using escaping (such as with the php function htmlentities) or the XML builders class to build trusted output.
$output->addHTML( '<form action="'.$action.'" method="post">' );
OutputPage->addWikiText()
For most output, you should be using this function. It's a bit of a black magic function: wikitext goes in, HTML comes out, and a whole lotta arcane code and demon summonings happen in between.
$output->addWikiText("This is some ''lovely'' [[wikitext]] that will '''get''' parsed nicely.");
What's worth noting is that the parser will view your chunks as cohesive wholes and paragraph accordingly. That is...
$output->addWikiText( '* Item 1' );
$output->addWikiText( '* Item 2' );
$output->addWikiText( '* Item 3' );
Will output three lists with one item each, which probably wasn't intended.
Note however, if you just want to insert a system message and have it treated like parsed wikitext, you can use code like $this->getOutput()->addHtml( $this->msg( 'key-of-message' )->parse() )
.
This will not have the issue with nested parser calls mentioned above.
workaround #1
Important: these work arounds are only needed if you are making a transcludable special page. Normal special pages do not need these.
As a workaround, you can have your extensions convert Wikitext to HTML using a separate Parser object and then use addHTML()
.
Example:
$wgOut->addHTML( $this->sandboxParse( "Here's some '''formatted''' text." ) );
# Assuming this is inside the same class as the rest of your special page code
function sandboxParse( $wikiText ) {
$myParser = new Parser();
$user = $this->getUser();
$title = self::getTitleFor('YourCanonicalSpecialPageName');
$myParserOptions = ParserOptions::newFromUser( $user );
$result = $myParser->parse( $wikiText, $title, $myParserOptions );
return $result->getText();
}
workaround #2
I tried the above, and found that the same problem now applied to any tags in the transcluded text. This won't be a problem for a lot of extensions, but the extension I was writing was intended to show wikitext from another page as part of its functionality, so this was a problem.
The process for parsing a page which transcludes a special page seems to be this:
- Replace {{Special:MyExtension}} with a UNIQ-QINU marker (because SpecialPage output is expected to be ready-to-output HTML)
- Replace any tags with QINU markers as above
- Parse everything else from wikitext to HTML
- Replace all QINU markers with their respective stored values, in a single pass
The process for parsing a page which transcludes a non-special page, though, is apparently like this:
- Replace {{:Normal Article Name}} or {{Template Name}} with contents of transcluded page (because transcluded pages contain unparsed wikitext)
- Replace any tags with QINU markers as above
- Parse everything else from wikitext to HTML
- Replace all QINU markers with their respective stored values, in a single pass
The problem is apparently that in the earlier case, the parsing of the SpecialPage's wiki text is lacking the final QINU decoding step (why?), so all the QINU markers are left undecoded. (This may be a leftover from using the same syntax to invoke transclusion of a wikitext page, which is just pasted straight into the host page's wikitext contents and parsed, as is used to invoke transclusion of a SpecialPage, which must not be parsed at all. Wherever the code is that decides "wait, this is a special page -- replace it with a QINU", it should be doing the extra unstripGeneral before doing the QINU substitution.)
So I just did the following -- after this line:
$htOut = $wgParser->recursiveTagParse( $iText );
...I added these lines (the second one is only because the function definition for the first one recommends it):
$htOut = $wgParser->mStripState->unstripGeneral( $htOut );
$htOut = $wgParser->mStripState->unstripNoWiki( $htOut );
Since I have now documented this, of course, I will now find a tragic flaw with it and feel really stupid... but as long as it seems to be working, I had to note it here. (It is also important to note the problem with work-around #1.) Also, I have only tested this with MediaWiki 1.10.1. The problem still exists under MW 1.14, but this solution may or may not work. --Woozle 18:26, 9 April 2009 (UTC)
OutputPage->showErrorPage()
An error page is shown.
The arguments $title
and $msg
specify keys into $this->msg(), not text.
An example:
$output->showErrorPage( 'error', 'badarticleerror' );
- 'error' refers to the text "Error".
- 'badarticleerror' refers to the text "This action cannot be performed on this page.".
You can also specify message objects or add parameters:
$output->showErrorPage( 'error', 'badarticleerror', [ 'param1', 'param2' ] );
$messageObject = new Message(...);
...
$output->showErrorPage( 'error', $messageObject );
$titleMessageObject = new Message(...);
$messageObject = new Message(...);
...
$output->showErrorPage( $titleMessageObject, $messageObject );
WebRequest.php
The WebRequest class is used to obtain information from the GET and POST arrays. Using this is recommended over directly accessing the superglobals. The WebRequest object is accessible from extensions by using the RequestContext .
Database.php
MediaWiki has a load of convenience functions and wrappers for interacting with the database, using the \Wikimedia\Rdbms\Database class.
It also has an interesting load balancing scheme in place.
It's recommended you use these wrappers.
Check out Database.php
for a complete listing of all the convenience functions, because these docs will only tell you about the non-obvious caveats.
See Manual:Database access .
User.php
The User class is used to represent users on the system.
SpecialPage->getUser() should be used to obtain a User object for the currently logged in user.
The use of the global $wgUser
is deprecated
Title.php
Title represents the name of a page in the wiki.
This is useful because MediaWiki does all sorts of fun escaping and special case logic to page names, so instead of rolling your own convert title to URL function, you create a Title object with your page name, and then use getLocalURL()
to get a URL to that page.
To get a title object for your special page from outside of the special page class, you can use SpecialPage::getTitleFor( 'YourCanonicalSpecialPageName' )
.
It will give you a localised title in the wiki's language.
Custom special pages
There are various ways to provide your own special pages not bundled within MediaWiki:
- One method is to install an extension that generates a form to create or edit an article. A list of extensions currently available, can be found at Category:Special page extensions .
- You can also write an extension which provides your own special page. Writing your own extension requires PHP coding skill and comfort with object oriented design and databases also is helpful. You will also need to know how to use code to create and edit MediaWiki articles. For more information, please see this discussion.
- You can also display a custom page through JavaScript, in place of the default error message "Unknown special page" (or the "This page is intentionally left blank." message, if using a subpage of Special:BlankPage). In MediaWiki:Common.js, check for wgPageName , then hide the MediaWiki-generated content (just appendCSS
{visibility:hidden;}
), and inject custom HTML (innerHTML
) into thedocument.getElementById('bodyContent')
ordocument.getElementById('mw_contentholder')
. For an example, see meta:User:Krinkle/Tools/Real-Time Recent Changes.
FAQ
Setting an Extension Title
MediaWiki does not set the title of the extension, which is the developer's job.
It will look for the name of the extension when Special:Specialpages is called or the special page is loaded.
In the function execute( $par ) section, use OutputPage methods to title the extension like: $this->getOutput()->setPageTitle("your title");
The place where the extension can be found (as specified by what is passed into the SpecialPage constructor) is the key--except that it is not capitalized because of getDescription()
, the internally used function that finds out the title (or, what they call description) of the special page, strtolower
the name.
"ThisIsACoolSpecialPage"'s key would be "thisisacoolspecialpage."
Theoretically, getDescription
can be overloaded in order to avoid interacting with the message cache but, as the source code states: "Derived classes can override this, but usually it is easier to keep the default behavior.
Furthermore, this prevents the MediaWiki namespace from overloading the message, as below.
Localizing the Extension Name
So you've just installed a shiny new MediaWiki extension and realize: "Oh no, my wiki is in French, but the page is showing up as English!"
Most people wouldn't care, but it's actually a quite simple task to fix (as long as the developer used the method explained on this page).
No noodling around in source code.
Let's say the name of the page is DirtyPages
and the name comes out to "List of Dirty Pages" but you want it to be (and excuse my poor French) "Liste de Pages Sales".
Well, it's as simple as this:
- Navigate to MediaWiki:DirtyPages, this page may not exist, but edit it anyway
- Insert "Liste de Pages Sales" and save
And voilà (pardon the pun), the change is applied.
This is also useful for customizing the title for your wiki within your language: for instance, the developer called it "List of Dirty Pages" but you don't like that name, so you rename it "List of Pages needing Cleanup". Check out Special:Allmessages to learn more.
Also, if your extension has a large block of text that does change, like a warning, don't directly output the text. Instead, add it to the message cache and when the time comes to output the text in your code, do this:
$wgOut->addWikiText( $this->msg( 'dirtypageshelp' ) );
Then this message too can be customized at MediaWiki:Dirtypageshelp.
See also Help:System message .
Restricting page access
Do not display your Special Page on Special:SpecialPages
Sometimes you may want to limit the visibility of your Special Page by removing it from Special:SpecialPages and making it visible to only those users with a particular right.
You can do this in the constructor by passing in a $restriction
parameter; e.g., “editinterface”, a right only assigned to sysops by default; see the User rights manual for other available user rights.
function __construct() {
parent::__construct( 'MyExtension', 'editinterface' ); // restrict to sysops
}
Or you can create your own right in the setup file and assign it to sysops, e.g.:
"AvailableRights": [
"myextension-right"
],
"GroupPermissions": {
"sysop": {
"myextension-right": true
}
}
and then call the constructor with your right:
function __construct() {
parent::__construct( 'MyExtension', 'myextension-right' );
}
Prevent access to your Special Page
Even if you restrict your page in the constructor, as mentioned above, it will still be viewable directly via the URL, e.g. at Special:MySpecialPage.
In order to actually limit access to your SpecialPage you must call $this->checkPermissions()
in the execute
method.
If you need more fine-grained control over permissions, you can override $this->checkPermissions()
, and/or add whatever permissions-checking is required for your extension.
Disabling Special:UserLogin and Special:UserLogout pages
In LocalSettings.php you can use the SpecialPage_initList hook to unset unwanted built-in special pages. See "making a few SpecialPages restricted" if you need conditional unsetting of special pages for example for certain user groups. The general message "You have requested an invalid special page." is shown if users try to access such unset special pages.
$wgHooks['SpecialPage_initList'][] = function ( &$list ) {
unset( $list['Userlogout'] );
unset( $list['Userlogin'] );
return true;
};
A different approach would be to use the DisabledSpecialPage callback. This approach may be preferred if you're only disabling the special page "temporarily", because the default message in this case would say: "This page has been disabled by a system administrator." instead of pretending the page does not exist at all. This gives clear hint that the page maybe activated at a later time.
$wgSpecialPages['Userlogout'] = DisabledSpecialPage::getCallback( 'Userlogout' );
$wgSpecialPages['Userlogin'] = DisabledSpecialPage::getCallback( 'Userlogin' );
It is also possible to add custom lengthy explanation of why you're disabling the special page, by giving a message key as the second argument of the callback. To do so first create a system message "MediaWiki:Userlogout-disable-reason" and write all the explanation there. The message will be parsed in a block format. Then in LocalSettings.php add:
$wgSpecialPages['Userlogout'] = DisabledSpecialPage::getCallback( 'Userlogout', 'Userlogout-disable-reason' );
Adding logs
On MediaWiki, all actions by users on wiki are tracked for transparency and collaboration. See Manual:Logging to Special:Log for how to do it.
Changing the groups on Special:Specialpages
If you're an extension developer, you have to implement the getGroupName()
method as described in the Special page group section of this page.
Since MediaWiki 1.21, the special page group can be overridden by editing a system message.
This method is not intended to be used by extension developers, but by site admins.
The group name must be placed in the specialpages-specialpagegroup-<special page name>
message, where <special page name>
is the canonical name (in english) of the special page in lowercase.
For example, if you want to set the group under which "Special:MyLittlePage" is displayed on Special:Specialpages to "MyLittleGroup", you just have to create "MediaWiki:Specialpages-specialpagegroup-mylittlepage" with content "MyLittleGroup".
"Special:MyLittlePage" will then show up under the group "MyLittleGroup", which you can name under "MediaWiki:Specialpages-group-mylittlegroup".
If you want to change the group of existing special pages, have a look on Special:SpecialPages&uselang=qqx and use those names instead of "mylittlepage".
Unlisting the page from Special:Specialpages
To remove a special page from the Special:Specialpages altogether, pass a false
as a third parameter to the SpecialPage parent constructor, as described in the SpecialPage Constructor section of this page.
If you need more complicated logic to determine whether the page should be listed or not, you can also override the isListed()
function, but using the constructor parameter is simpler.
Getting a list of special pages and their aliases on a wiki
Simply use the "siteinfo" API module to retrieve the information from the wiki like e.g. /api.php?action=query&meta=siteinfo&siprop=specialpagealiases.
See also
- HTMLForm – Tutorial on creating checkboxes, text areas, radio buttons, etc. in special pages